Este modulo permite llevar el registro de la fecha y hora una vez establecidas en su memoria.
Una explicación del uso de los pins se encuentra aquí. Como recargar la batería, porque ahora (Sept 2015) me doy cuenta de que no conserva la información previa. Es factible usar el pin BAT para monitorear el voltaje, pero como cargarla.
Comentario en el foro de Arduino hecho por.
jremington
The charging circuit only works if you connect Vcc on the module to 5 V. Looking at the schematic, I suspect that if the module is powered for a long time at 5V, this particular circuit will overcharge and eventually destroy the cell. The charging rules for the LIR2032 can be found here: http://www.powerstream.com/p/Lir2032.pdf
How to check battery level on a RTC DS1307 ?
En la primera parte vamos a generar un sketch con el cual se puede inicializar las variables hora minuto segundo, día mes año.
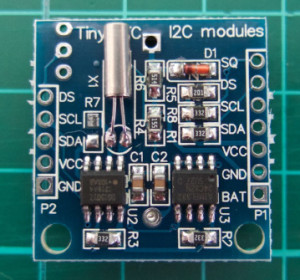
#include "Wire.h" #define DS1307_ADDRESS 0x68 byte zero = 0x00; //workaround for issue #527 void setup(){ Wire.begin(); Serial.begin(9600); setDateTime(); //MUST CONFIGURE IN FUNCTION } void loop(){ printDate(); delay(1000); } void setDateTime(){ byte second = 0; //0-59 byte minute = 51; //0-59 byte hour = 21; //0-23 byte weekDay = 2; //1-7 byte monthDay = 20; //1-31 byte month = 4; //1-12 byte year = 15; //0-99 Wire.beginTransmission(DS1307_ADDRESS); Wire.write(zero); //stop Oscillator Wire.write(decToBcd(second)); Wire.write(decToBcd(minute)); Wire.write(decToBcd(hour)); Wire.write(decToBcd(weekDay)); Wire.write(decToBcd(monthDay)); Wire.write(decToBcd(month)); Wire.write(decToBcd(year)); Wire.write(zero); //start Wire.endTransmission(); } byte decToBcd(byte val){ // Convert normal decimal numbers to binary coded decimal return ( (val/10*16) + (val%10) ); } byte bcdToDec(byte val) { // Convert binary coded decimal to normal decimal numbers return ( (val/16*10) + (val%16) ); } void printDate(){ // Reset the register pointer Wire.beginTransmission(DS1307_ADDRESS); Wire.write(zero); Wire.endTransmission(); Wire.requestFrom(DS1307_ADDRESS, 7); int second = bcdToDec(Wire.read()); int minute = bcdToDec(Wire.read()); int hour = bcdToDec(Wire.read() & 0b111111); //24 hour time int weekDay = bcdToDec(Wire.read()); //0-6 -> sunday - Saturday int monthDay = bcdToDec(Wire.read()); int month = bcdToDec(Wire.read()); int year = bcdToDec(Wire.read()); //print the date EG 3/1/11 23:59:59 Serial.print(month); Serial.print("/"); Serial.print(monthDay); Serial.print("/"); Serial.print(year); Serial.print(" "); Serial.print(hour); Serial.print(":"); Serial.print(minute); Serial.print(":"); Serial.println(second); Serial.print(" "); Serial.println(weekDay); }
Ahora combinando monitor serial y LCD.
// #include "LiquidCrystal_I2C.h" #include "Wire.h" #define DS1307_ADDRESS 0x68 LiquidCrystal_I2C lcd(0x3F,20,4); void setup(){ Wire.begin(); lcd.backlight(); lcd.init(); Serial.begin(9600); } void loop(){ printDate(); delay(1000); } byte bcdToDec(byte val) { // Convert binary coded decimal to normal decimal numbers return ( (val/16*10) + (val%16) ); } void printDate(){ // Reset the register pointer Wire.beginTransmission(DS1307_ADDRESS); byte zero = 0x00; Wire.write(zero); Wire.endTransmission(); Wire.requestFrom(DS1307_ADDRESS, 7); int second = bcdToDec(Wire.read()); int minute = bcdToDec(Wire.read()); int hour = bcdToDec(Wire.read() & 0b111111); //24 hour time int weekDay = bcdToDec(Wire.read()); //0-6 -> sunday - Saturday int monthDay = bcdToDec(Wire.read()); int month = bcdToDec(Wire.read()); int year = bcdToDec(Wire.read()); //print the date (Serial Monitor and LCD Screen EG 3/1/11 23:59:59 Serial.print(month); Serial.print("/"); Serial.print(monthDay); Serial.print("/"); Serial.print(year); Serial.print(" "); Serial.print(hour); Serial.print(":"); Serial.print(minute); Serial.print(":"); Serial.println(second); switch(weekDay){ case 1: Serial.println("Domingo"); break; case 2: Serial.println("Lunes"); break; case 3: Serial.println("Martes"); break; case 4: Serial.println("Miercoles"); break; case 5: Serial.println("Jueves"); break; case 6: Serial.println("Viernes"); break; case 7: Serial.println("Sabado"); break; } lcd.clear(); lcd.setCursor(3,0); lcd.print(month); lcd.print("/"); lcd.print(monthDay); lcd.print("/"); lcd.print(year); lcd.setCursor(3,1); lcd.print(hour); lcd.print(":"); lcd.print(minute); lcd.print(":"); lcd.print(second); lcd.setCursor(3,3); lcd.print("Hoy es:"); lcd.setCursor(11,3); switch(weekDay){ case 1: lcd.print("Domingo"); break; case 2: lcd.print("Lunes"); break; case 3: lcd.print("Martes"); break; case 4: lcd.print("Miercoles"); break; case 5: lcd.print("Jueves"); break; case 6: lcd.print("Viernes"); break; case 7: lcd.print("Sabado"); break; } }
Incluyamos un sensor de temperatura DS1307 eliminando el monitor serial.
// #include "DHT.h" #include "LiquidCrystal_I2C.h" #include "Wire.h" #define DS1307_ADDRESS 0x68 #define DHTPIN 2 // El pin 2 del sensor se conecta al pin 2 de las entradas digitales de Arduino uno. #define DHTTYPE DHT11 LiquidCrystal_I2C lcd(0x3F,20,4); DHT dht(DHTPIN, DHTTYPE); void setup(){ Wire.begin(); lcd.backlight(); lcd.init(); dht.begin(); } void loop(){ printDate(); tempHumid(); delay(1000); } byte bcdToDec(byte val) { // Convert binary coded decimal to normal decimal numbers return ( (val/16*10) + (val%16) ); } void tempHumid(){ lcd.setCursor(0,1); lcd.print("Humedad: "); lcd.setCursor(0,2); lcd.print("Temperatura:"); // Espera unos segundos entre lecturas. delay(1000); // La lectura de la temperatura y humedad toma alrededor de 250 milisegundos! // Las lecturas del sensor pueden estar el alto por 2 segundos ‘old’ (esto ya que es un //procesador muy lento) float h = dht.readHumidity(); // Lectura de temperature en Celsius float t = dht.readTemperature(); // Lectura de temperatura en Fahrenheit float f = dht.readTemperature(true); // Verifica si las lecturas fueron correctas o se dio un timeout (intenta nuevamente). if (isnan(h) || isnan(t) || isnan(f)) { lcd.clear(); lcd.print("No se obtuvieron valores adecuados del sensor DHT!"); return; } lcd.setCursor(12,1); lcd.print(h); lcd.print(" %"); lcd.setCursor(12,2); lcd.print(t); lcd.print(" *C"); lcd.setCursor(12,3); lcd.print(f); lcd.print(" *F"); } void printDate(){ // Reset the register pointer Wire.beginTransmission(DS1307_ADDRESS); byte zero = 0x00; Wire.write(zero); Wire.endTransmission(); Wire.requestFrom(DS1307_ADDRESS, 7); int second = bcdToDec(Wire.read()); int minute = bcdToDec(Wire.read()); int hour = bcdToDec(Wire.read() & 0b111111); //24 hour time int weekDay = bcdToDec(Wire.read()); //0-6 -> sunday - Saturday int monthDay = bcdToDec(Wire.read()); int month = bcdToDec(Wire.read()); int year = bcdToDec(Wire.read()); lcd.clear(); lcd.setCursor(0,0); lcd.print(month); lcd.print("/"); lcd.print(monthDay); lcd.print("/"); lcd.print(year); lcd.setCursor(12,0); lcd.print(hour); lcd.print(":"); lcd.print(minute); lcd.print(":"); lcd.print(second); lcd.setCursor(0,3); switch(weekDay){ case 1: lcd.print("Domingo"); break; case 2: lcd.print("Lunes"); break; case 3: lcd.print("Martes"); break; case 4: lcd.print("Miercoles"); break; case 5: lcd.print("Jueves"); break; case 6: lcd.print("Viernes"); break; case 7: lcd.print("Sabado"); break; } }
Sensor humedad y RTC
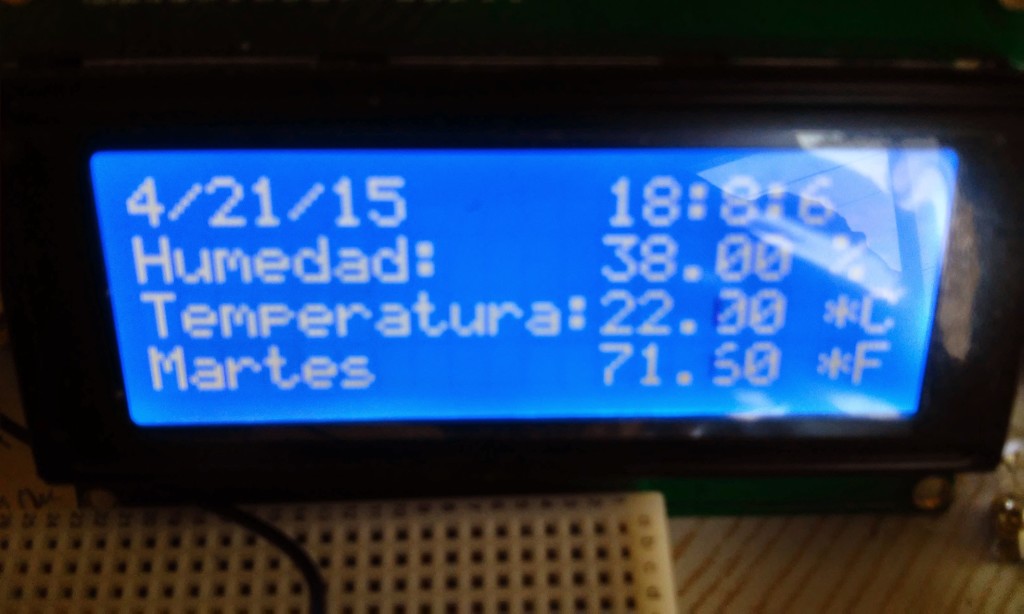
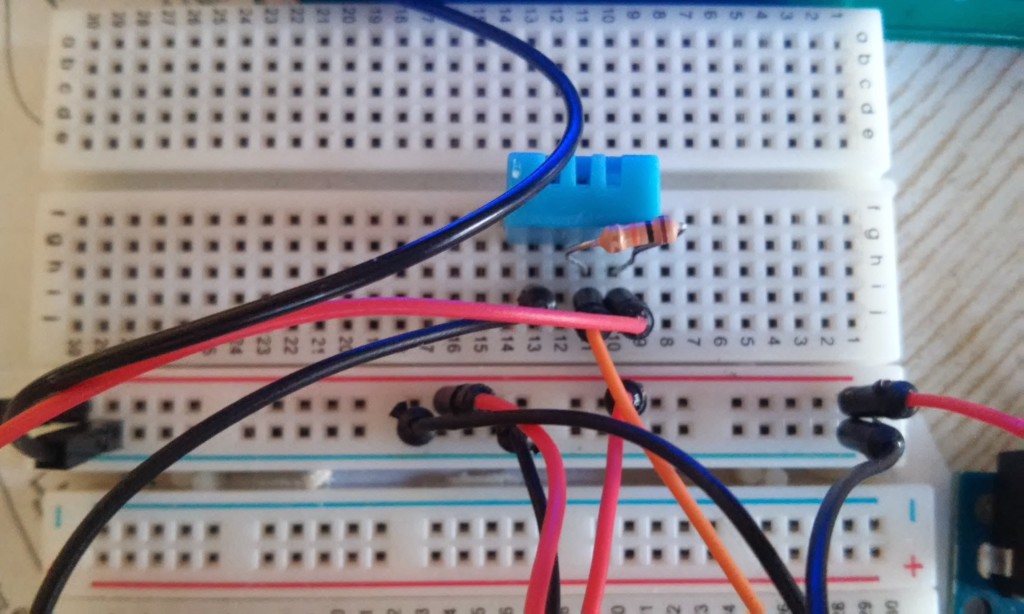
Revisando otros sketches me encontré con este en el que se usan instrucciones para importar la hora y fecha de la computadora con la cual se transfiere al ARDUINO.
/* Titulo: Ajuste Fecha Hora a CPU Autor: Jorge Luis Herrera Fecha: 3 Jul 2015 Version: A */ #include <LiquidCrystal_I2C.h> #include <Wire.h> #include "RTClib.h" LiquidCrystal_I2C lcd(0x3F,20,4); //Real Time Clock RTC_DS1307 RTC; void setup(){ Serial.begin(9600); lcd.backlight(); lcd.init(); Wire.begin(); RTC.begin(); RTC.adjust(DateTime(__DATE__, __TIME__)); } void loop(){ //Desplegar Fecha y Hora DateTime now = RTC.now(); //FECHA //Dia lcd.setCursor(0,1); lcd.print(now.day(), DEC); lcd.print('/'); //Mes lcd.print(now.month(), DEC); lcd.print('/'); //Ano lcd.print(now.year(), DEC); lcd.print(' '); //HORA lcd.setCursor(10,1); lcd.print(now.hour(), DEC); lcd.print(':'); lcd.setCursor(13,1); lcd.print(now.minute(), DEC); lcd.print(':'); lcd.print(now.second(), DEC); delay(1000); }
Mucho más compacto y asegurando una sincronía adecuada.
La pagina de Adafruit para instalar la biblioteca RTClib